Let’s see how to convert char to string in Python.
Asume given char list is :
Char : ['m', 'y', 'c', 'h', 'a', 'r']
And we would like to convert it to:
String : mychar
There are a few ways to do that:
The code
The easiest method is to create a empty string, iterate the char and add char items to the string.
char = ['m', 'y', 'c', 'h', 'a', 'r'] def charToString(char): string = "" for x in char: string += x return string print(charToString(char))
Alternative method
There is an other method. You can use join function to join char into string.
i = ['m', 'y', 'c', 'h', 'a', 'r'] def chartostring(i): string = "" return string.join(i) print(chartostring(i))
As you may notice .join() function is making chars a one string.
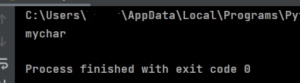
Similarly you are able to do the same by .tostring() function. You may think this looks uglier but in fact tostring() is faster than .join() in this case.
Keeping performance in mind this method is recommended. Hope now you know how to convert char to string with Python.