Python Turtle is a Python library that allows you to create 2D graphics. It is a great way to learn the basics of programming and to create simple animations.
We will learn how to create a wheel in Python Turtle.
How to create a wheel?
To draw a simple wheel, just use the below code:
import turtle turtle.circle(100) turtle.mainloop()
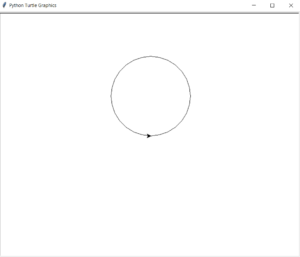
But let’s have even more fun and draw more wheels.
How to create two wheels?
This code will draw two wheels.
import turtle turtle.circle(100) turtle.circle(-100) turtle.mainloop()
To draw a wheel backwards, just use the below code. It draws backwards thanks to using -360 as a second argument in the circle function. By lowering the 360 number, you can draw only a part of the wheel.
import turtle turtle.circle(100, -360) turtle.mainloop()
How to create a dotted line wheel?
You can also create a dotted line wheel by using the penup and pendown functions. The penup function raises the pen, so that it does not draw anything when it moves. The pendown function lowers the pen, so that it starts drawing again.
import turtle for i in range(12): turtle.circle(100, 15) turtle.penup() turtle.circle(100, 15) turtle.pendown() turtle.mainloop()
I used a simple for loop and penup and pendown functions.
How to create a black wheel?
The next wheel will be filled in. This is the black wheel.
import turtle turtle.begin_fill() turtle.circle(100) turtle.end_fill() turtle.mainloop()
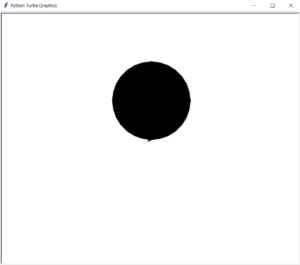
How to create a purple wheel?
Why not draw a black one? Let’s change the color! This is the purple wheel.
import turtle turtle.fillcolor('purple') turtle.begin_fill() turtle.circle(100) turtle.end_fill() turtle.mainloop()
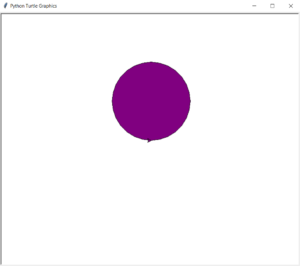
Draw a wheel with a border
Of course, you can use another color. The code is self explanatory. Just write the other color into the Python code.
Oh, you haven’t just changed your mind? What do you mean you like the black one and you also like purple? I have an idea! Let’s add a border to the wheel and make it purple with a black border.
import turtle turtle.pensize(20) turtle.color('black') turtle.fillcolor('purple') turtle.begin_fill() turtle.circle(100) turtle.end_fill() turtle.mainloop()
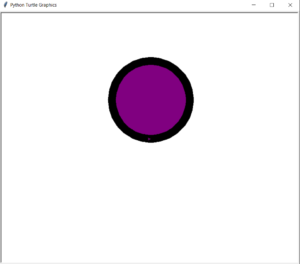
Creating a Spinning Wheel
We will learn how to create a spinning wheel in Python Turtle.
To do this, we will use the for loop to repeatedly draw the wheel. We will also use the delay function to slow down the animation.
The following code will create a spinning wheel with a radius of 100 pixels:
import turtle for i in range(100): turtle.circle(100, 360) turtle.delay(10)
You can adjust the speed of the animation by changing the value of the delay function. A smaller value will make the animation faster, and a larger value will make the animation slower.
You can also change the color of the wheel by using the fillcolor function. The following code will create a spinning wheel that changes color every 10 frames:
import turtle colors = ['red', 'green', 'blue', 'purple'] for i in range(100): turtle.fillcolor(colors[i % len(colors)]) turtle.circle(100, 360) turtle.delay(10)
Creating a Moving Wheel
To do this, we will use the while loop to repeatedly draw the wheel. We will also use the goto function to move the turtle to a new location.
The following code will create a moving wheel that starts at the center of the screen and moves to the right:
import turtle x = 0 y = 0 while True: turtle.goto(x, y) turtle.circle(100, 360) x += 10 if x >= 400: x = 0
You can adjust the speed of the wheel by changing the value of the x variable. A larger value will make the wheel move faster, and a smaller value will make the wheel move slower.
You can also change the direction of the wheel by changing the sign of the x variable. A positive value will make the wheel move to the right, and a negative value will make the wheel move to the left.
Now you know how to create a wheel with Python Turtle. Please check more Python Turle drawnings.