Let’s see how to handle unit conversion in Python. I’m taking cm to inch conversion as an example.
Python, with its clear syntax and numerical capabilities, provides an excellent environment for performing these conversions.
Centimeter to Inch Conversion in Python
CM_IN_INCH = 2.54 def cm_in_inch(cm): return cm / CM_IN_INCH number_cm = float(input("How many cm do you want to convert to inch?")) print("Entered number of %s centimeters equals %s inches" % (number_cm, round(cm_in_inch(number_cm), 2)))
Conversion code explanation
First, I defined the CM_IN_INCH constant which I will definitely use later.
Next, I defined a simple function that performs the conversion from cm to inches.
Then, I take input from the user.
And finally printing the result rounded to the two decimal places.
The result is as follows:
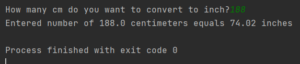
Getting User Input and Displaying Output
To make the script interactive, we prompt the user to enter the length in centimeters using the input()
function. The input is then converted to a floating-point number using float()
to handle decimal values. It’s good practice to include input validation to handle cases where the user might enter non-numeric input. For simplicity, input validation is omitted in this basic example but should be considered for more robust applications.
The converted value in inches is then calculated by calling the cm_in_inch()
function. The round()
function is used to round the result to two decimal places for clearer output. While we’ve rounded to two decimal places here, the desired precision might vary depending on the application. You could make the number of decimal places a parameter to the function or use string formatting for more control over the output precision.
Finally, the original centimeter value and the calculated inch value are displayed to the user using an f-string for formatted output, clearly stating the units for both values.